Welcome back to our guided series where we are arming you with the tools and knowledge to create a Power App integrated with Python scripts to forecast sales leveraging data from Microsoft Dynamics Business Central. In this installation of the series, we are diving deep into the realms of Python script creation and setting up an Azure Function App.
A vital step in our project is setting up an environment where our Python script can effectively communicate with the Power App, essentially acting as the bridge between the data and the predictive analysis tool we are aiming to create. Publishing Python scripts as Azure function apps and exposing them as APIs facilitates this communication, allowing the smooth transfer of data for analysis.
Our focus in this chapter is to walk you through the meticulous process of:
Setting up your development environment with Visual Studio Code and necessary extensions.
Crafting a Python function that leverages the scipy and matplotlib libraries to perform linear regression on sales data to derive the slope and intercept, essential components for predicting future sales.
Developing and deploying your function app in Azure, a process that puts your Python script into action, making it accessible and usable as a functioning API.
Implementing and managing your API through Azure's intuitive API management service, ensuring secure and efficient operation.
Testing the setup rigorously using tools like Postman to ensure the Python script is receiving and processing the data correctly, returning the desired output.
We begin our journey with setting up Visual Studio Code, installing Azure Functions Core Tools, and embedding necessary extensions that will form the backbone of our project.
As we progress through the blog, we delve into the intricacies of creating a function both in the workspace and Azure, a guided pathway directing you at each juncture, from selecting Python as the language to determining authorization levels.
In the subsequent sections, we impart the knowledge of embedding your new Python code into the __init__.py file, a script crafted meticulously to accept Business Central Item Ledger history and return both the slope and intercept, the pivotal elements in predicting future sales.
With a comprehensive guide on deploying to Function App and a detailed walkthrough on testing your Function App using Postman, we ensure that you are well-equipped to handle and validate your setup, fostering confidence in the process.
The final leg of our journey in this blog unravels the essentials of API management and subscriptions in Azure, setting a stage for the upcoming blog where the API key generated here will play a pivotal role.
Whether you are a novice stepping into the world of Azure Function Apps or a seasoned developer looking to enhance your skill set, this blog serves as a meticulous guide, walking you through each step with precision, ensuring a holistic understanding and a successful setup, ready for integration with Power Apps in our upcoming blogs.
Join us as we unlock the potential of Python scripts in Azure Function Apps, paving the path for predictive analytics in sales, a journey from raw data to insightful forecasts, step by empowered step.
The Steps
1. Visual Studio Code. Install Visual Studio Code.
2. Azure Functions Core Tools. Install the Azure functions core Tools.
3. Extensions. Install Azure Functions in Visual Studio Code. Click on Extensions in the left navigation pane and then search for and install Azure Functions. The system will automatically install:
a. Azure Functions
b. Azure Account
c. Azure Resources
4. Sign into Azure. Sign into Azure by first clicking on the Azure button in the left navigation pane and then click Sign in to Azure...
5. Create Function in Workspace. Click on the Workspace Create Function button that looks like a lightening Bolt. Then:
a. Identify a folder that you will use and then select Python as the language.
b. Click on Skip virtual environment.
c. Then click on HTTP trigger for the first function.
d. Give your function a name.
e. Select Function as the Authorization level.
f. The click on Open in current window.
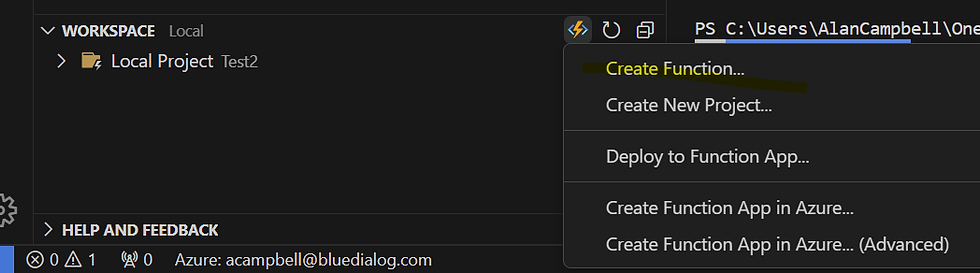
6. Create Function in Azure. Right click on Function App and click on Create Function App in Azure. Give the Function App a name. Then:
a. Select a version of Python that you want to use.
b. Select a resource group or create a new one.
c. Select a Location.
d. Select Consumption as the hosting plan.
e. Select or create a storage account.
f. Create a new Application Insights.
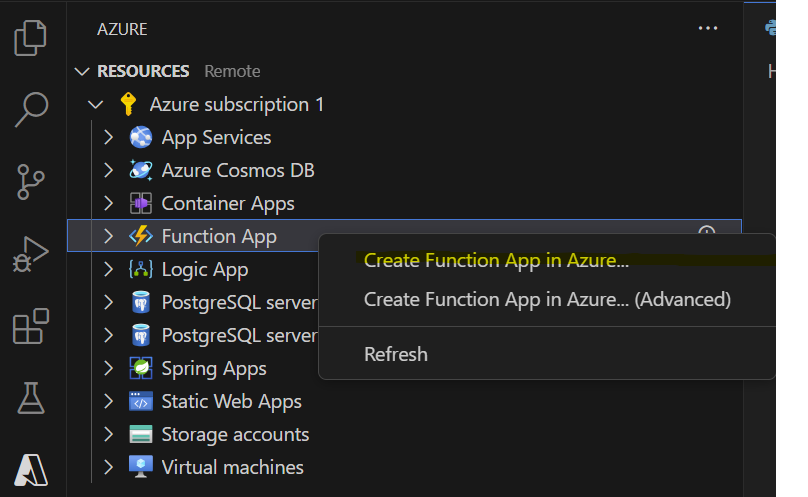
7. Apply New Python Code. The next step is to copy and paste the code below into _init_.py replacing the existing code. This is the Python script that will accept Business Central Item Ledger history and return both the Slope and Intercept.
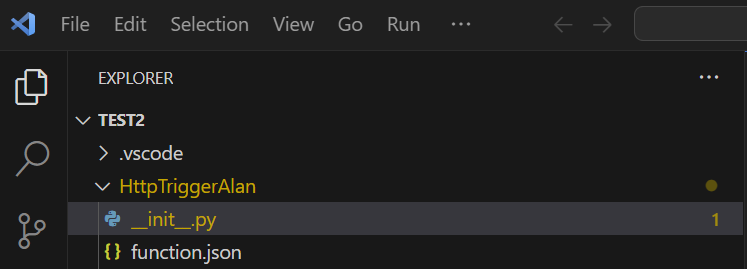
import json
import azure.functions as func
from scipy import stats
def main(req: func.HttpRequest) -> func.HttpResponse:
try:
body = req.get_json()
# Get the X and Y strings
x_str = body.get('x')
y_str = body.get('y')
# Extract the x and y lists
try:
x = list(map(int, x_str.split(',')))
y = list(map(int, y_str.split(',')))
except ValueError:
return func.HttpResponse(
json.dumps({"error": "Invalid integer in array"}),
status_code=400
)
# Get the stats for x and y
slope, intercept, r, p, std_err = stats.linregress(x, y)
# normal return
response_data = {
'slope':slope,
'intercept':intercept,
'r':r,
'p':p,
'std_err':std_err
}
return func.HttpResponse(
json.dumps(response_data),
mimetype="application/json",
status_code=200
)
except Exception as e:
return func.HttpResponse(
json.dumps({"error": str(e)}),
mimetype="application/json",
status_code=500
)
8. Deploy to Function App. Click on Azure in the left navigation pane. Then right mouse on your app under the Function App line. Click on Deploy to Function App... You will then receive a pop-up where you once again click on the Deploy button.
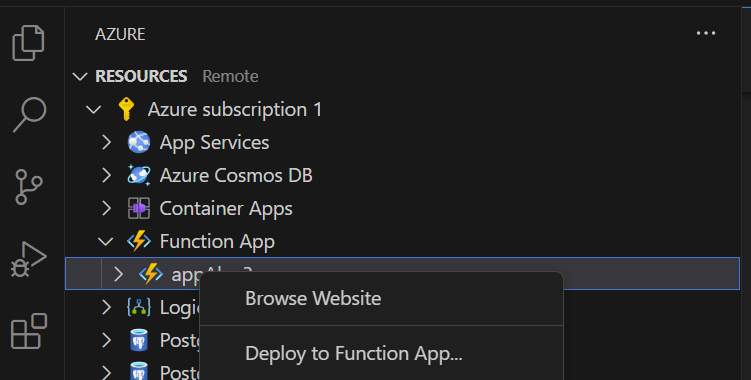
9. Your Function App in Azure
a. Go to https://portal.azure.com/
b. Search for Function App. You should now see your Function App listed
c. Click on your function app
d. You should now see the Function App listed in the right pane. Click on it.
e. Click on Code + Test in the left navigation pane. You should now see your code.
f. Click on Get function URL in the header and copy the value.
10. Testing Your Function App. I like to use Postman to test.
a. Add a new request in Postman.
b. Select POST and paste in the URL that you copied in the Azure.
c. In the Body section click on raw.
d. Paste the following JSON in the Body and click on Send
{
"x": "0,1,2,3,4,5,6,7,8,9,10,11,12,13,14",
"y": "4535,3887,6477,4534,6477,3239,5182,5182,2592,3888,1944,3239,5829,3886,4000"
}
e. Your response should look like this:
{
"slope": -96.64999999999999,
"intercept": 5002.616666666667,
"r": -0.3247983548185419,
"p": 0.2375335363376549,
"std_err": 78.05630651766381
}
11. API Management. Next we will setup the API in Azure.
a. In your Function App left navigation pane you will see an API section about 1/2 of the way down the list. Click on API Management.
b. Click on -- Create New -- in the right pane.
c. Select your function app and answer the questions as appropriate.
d. Click on Export. This may take up to 30 minutes to complete.
12. Testing Your API
a. In the header search for Function App and then click on your app.
b. Click on API Management in the left navigation pane.
c. Click on Go to API Management in the header.
d. Click on APIs under the API section in the left navigation pane.
e. Click on your API name under All APIS in the center pane.
f. Click on the POST operation
g. Click on Test in the banner
h. Enter the following:
- Select Content-Type for the NAME
- Select application/json for the VALUE
- In the Request Body select Raw and enter the same JSON that you entered for the last test
i. Click on Send. You should get a 200 response and the same information as you did in the previous test.
13. Subscriptions. You will need an API Key in the next blog.
a. click on Subscriptions under APIs in the left navigation pane.
b. Add a subscription and name it ApiKey.
Conclusion
Congratulations on successfully navigating through the comprehensive guide we presented in this installment of our series! You are now one step closer to building a robust Power App integrated with Python scripts, fueled by data from Microsoft Dynamics Business Central.
In this chapter, we explored the profound depths of Python script creation and Azure Function App setup, crafting a pathway that connects your Power App with powerful predictive analytics tools. By leveraging the strengths of Python along with Azure's rich ecosystem, we are gradually bringing to life a tool that stands to revolutionize sales forecasting.
Let's quickly recap the significant milestones you achieved in this leg of the journey:
Initial Setup: Setting up your Visual Studio Code with the requisite tools and extensions, thereby laying a firm foundation for the project.
Python Function Creation: Crafting a Python function capable of performing linear regression to facilitate future sales predictions using scipy and matplotlib libraries.
Azure Function App: Developing and deploying your function app in Azure to breathe life into your Python script, making it a functioning API.
API Management and Testing: Learning to manage your API using Azure’s intuitive API management service, followed by rigorous testing to ensure its flawless functionality.
As we navigated through each section, we carried the ethos of empowering you with a clear understanding of each process, ensuring not just theoretical knowledge but also a hands-on experience that will stand you in good stead in the practical field.
Now, you have at your disposal a fully functioning Python script ready to be integrated with a Power App. You learned how to expose Python scripts as APIs, a vital procedure that promises seamless data transmission, setting a strong precedent for the communication between your predictive tool and the existing database.
Looking Ahead
As we draw curtains on this episode, it is essential to understand that this blog series is more than just a guide; it is your roadmap to unleashing the untapped potential that lies in integrating Python scripts with Power Apps.
In upcoming blogs in this series, we will steer towards the final lap of our course where we shall delve into:
Power Apps Integration: Understanding how to integrate your newly created API with Power Apps, leveraging the API key we generated in this chapter.
UI Elements: Introducing you to the various UI elements that will aid in presenting the data articulately in Power Apps.
Data Utilization: Demonstrating the utilization of slope and intercept values in crafting insightful sales forecasts.
Keep your API key secure as it will play a pivotal role in the upcoming blog. Armed with the knowledge and the tools you have gathered so far, you are all set to venture into the exciting world of Power Apps, bringing you closer to a tool that promises to be a game-changer in predictive sales analytics.
Join Us
Join us in the next blog as we continue to unravel the transformative potential that this integration promises, guiding you step by empowering step towards crafting an app that stands to redefine sales forecasting through the powerful amalgamation of Power Apps, Python scripts, and Microsoft Dynamics Business Central.
Thank you for being a part of this journey with us. Until next time, keep exploring, keep learning, and keep growing.
Comments